1)
Setup
and hold checks for paths launching from positive edge-triggered flip-flop and
being captured at positive edge-triggered flip-flop (rise-to-rise checks): Figure 1 shows a
path being launched from a positive edge-triggered flop and being captured on a
positive edge-triggered flop. In this case, setup check is on the next rising
edge and hold check is on the same edge corresponding to the clock edge on
which launching flop is launching the data.
 |
Figure 1 : Timing path from positive
edge flop to positive edge flop (rise to rise path)
|
Figure 2 below shows the setup and hold checks for positive edge-triggered register to positive edge-triggered register in the form of waveform. As is shown,
setup check occurs at the next rising edge and hold check occurs at the same
edge corresponding to the launch clock edge. For this case setup timing equation cab be given as:
Tck->q
+ Tprop + Tsetup < Tperiod + Tskew (for setup check)
And the equation for hold timing can be given as:
Tck->q
+ Tprop > Thold + Tskew (for
hold check)
Where
Tck->q : Clock-to-output delay of launch register
Tprop : Maximum delay of the combinational path between launch and capture register
Thold : Hold time requirement of capturing register
Tskew : skew between the two registers (Clock arrival at capture register - Clock arrival at launch register)
Also, we show below the
data valid and invalid windows. From this figure,
Data valid window = Clock period
– Setup window – Hold window
Start of data valid window = Tlaunch
+ Thold
End of data valid window = Tlaunch
+ Tperiod – Tsetup
In other words, data at the input of capture register can toggle any time between (Tlaunch + Thold) and (Tlaunch + Tperiod – Tsetup).
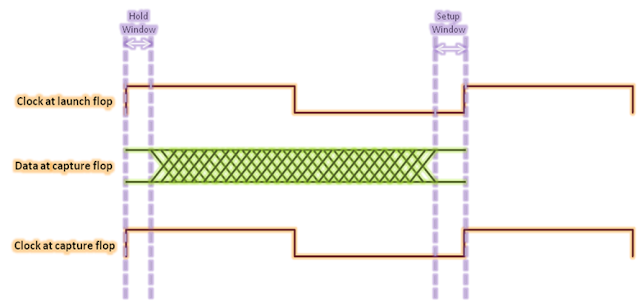 |
Figure 3: Figure showing data valid
window for rise-to-rise path
|
2) Setup
and hold checks for paths launching from positive edge-triggered flip-flop and
being captured at negative edge-triggered flip-flop: In this case, both
setup and hold check are half cycle checks; setup being checked on the next
falling edge at the capture flop and hold on the previous falling edge of clock
at the capture flop (data is launched at rising edge). Thus, with respect to
(case 1) above, setup check has become tight and hold check has relaxed.
 |
Figure 4: Timing path from positive
edge flop to negative edge flop (Rise-to-fall path)
|
Figure 5 below shows the setup and hold checks
in the form of waveforms. As is shown, setup check occurs at the next falling edge
and hold check occurs at the previous falling edge corresponding to the launch
clock edge. The equation for setup check can be written, in this case, as:
Tck->q
+ Tprop + Tsetup < (Tperiod/2) + Tskew (for setup check)
And the equation for hold check can be written as:
Tck->q
+ Tprop + (Tperiod/2) > Thold + Tskew (for
hold check)
 |
Figure 5: Setup and hold checks for
rise-to-fall paths
|
Also, we show below the
data valid and invalid windows. From this figure,
Data valid window = Clock period
– Setup window – Hold window
Start of data valid window = Tlaunch
– (Tperiod/2)+ Thold
End of data valid window = Tlaunch
+ (Tperiod/2) – Tsetup
As we can see, the data valid window is spread evenly
on both sides of launch clock edge.
 |
Figure 6: Figure showing data valid
window for rise-to-fall path
|
3)
Setup
and hold checks for paths launching from negative edge-triggered flip-flop and
being captured at positive edge-triggered flip-flop (rise-to-fall paths):
This case is similar to case 2; i.e. both setup and hold checks are half cycle
checks. Data is launched on negative edge of the clock, setup is checked on the
next rising edge and hold on previous rising edge of the clock.
 |
Figure 7: Timing path from negative
edge flop to positive edge flop (fall-to-rise path)
|
Figure
8 below shows the setup and hold checks in the form of waveforms. As is shown,
setup check occurs at the next rising edge and hold check occurs at the
previous rising edge corresponding to the launch clock edge. The equation for setup check can be written, in this case, as:
Tck->q + Tprop + Tsetup < (Tperiod/2) + Tskew (for setup check)
And the equation for hold check can be written as:
Tck->q + Tprop + (Tperiod/2) > Thold + Tskew (for hold check)
 |
Figure 8: Setup and hold checks for
fall to rise paths
|
Also, we show below the
data valid and invalid windows. From this figure,
Data valid window = Clock
period – Setup window – Hold window
Start of data valid window = Tlaunch
– (Tperiod/2)+ Thold
End of data valid window = Tlaunch
+ (Tperiod/2) – Tsetup
In this case too, data valid window spreads evenly on
both the sides of launch clock edge.
 |
Figure 9: Figure showing data valid
window for fall-to-rise path
|
4)
Setup
and hold checks for paths launching from negative edge-triggered flip-flop and
being captured at negative edge-triggered flip-flop (fall-to-fall paths):
The interpretation of this case is similar to case 1. Both launch and
capture of data happen at negative edge of the clock. Figure 10 shows a path
being launched from a negative edge-triggered flop and being captured on a
negative edge-triggered flop. In this case, setup check is on the next falling
edge and hold check is on the same edge corresponding to the clock edge on
which launching flop is launching the data.
 |
Figure 10: Path from negative edge flop
to negative edge flop (fall to fall path)
|
Figure
below shows the setup and hold checks in the form of waveforms. As is shown,
setup check occurs at the next falling edge and hold check occurs at the same
edge corresponding to the launch clock edge.
The equation for setup check can be given as:
Tck->q
+ Tprop + Tsetup < Tperiod + Tskew (for setup check)
And the equation for hold check can be given as:
Tck->q + Tprop > Thold
+ Tskew (for
hold check)
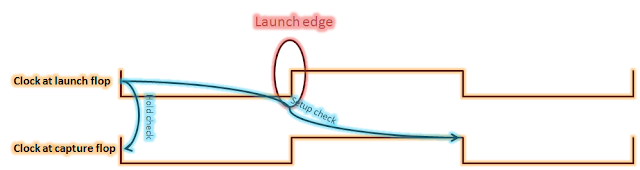 |
Figure 11: Setup and hold check for fall-to-fall path
|
Also, we show below the
data valid and invalid windows. From this figure,
Data valid window = Clock period
– Setup window – Hold window
Start of data valid window = Tlaunch
+ Thold
End of data valid window = Tlaunch
+ Tperiod – Tsetup
 |
Figure 12: Figure showing data valid
window for fall-to-fall path
|